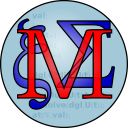 |
wxMaxima
|
41 explicit Svgout(
Configuration **configuration,
const wxString &filename = {},
double scale = 1.0);
43 const wxString &filename = {},
double scale = 1.0);
51 wxSize
Render(std::unique_ptr<Cell> &&tree);
53 wxSize GetSize()
const {
return m_size; }
54 bool IsOk()
const {
return m_isOk; }
63 std::unique_ptr<Cell> m_tree;
65 wxSVGFileDC m_recalculationDc;
66 wxSize m_size = wxDefaultSize;
bool ToClipboard()
Copies the svg representation of the list of cells that was passed to SetData()
Definition: SVGout.cpp:107
Svgout(Configuration **configuration, const wxString &filename={}, double scale=1.0)
Definition: SVGout.cpp:38
Definition: OutCommon.h:42
Definition: Configuration.h:83
wxSize Render(std::unique_ptr< Cell > &&tree)
Definition: SVGout.cpp:68
std::unique_ptr< wxCustomDataObject > GetDataObject()
Returns the svg representation in a format that can be placed on the clipBoard.
Definition: SVGout.cpp:102